Computational Engineering 101 – Part 5: Algorithms
Algorithms
In Part 4, we introduced the concept of Object Oriented Programming and stressed the importance of Objects for any Computational Engineer. In this post, we will learn how to use engineer algorithms with objects. As discussed in part 1, to be a Computational Engineer implies already that you are good engineer. An engineer knows how to apply various forms of knowledge to solve problems. You are probably used to engineering the ‘thing’, whatever that happens to be – a road, a duct, a city, etc. Now with an appreciation of objects under your belt, you should have all the skills you need to engineer algorithms which will help you unleash your engineering capabilities by creating the ‘thing that creates the thing’, not the ‘thing’.
Algorithms Use Objects
So why did we focus so much in that last post on Objects? An easy way to think of algorithms is to think of them as a function which for a certain input, will give you a certain output. In mathematical notation, we usually see the following: f(x) = y. That is – the function ‘f’ when fed the input ‘x’, will give you ‘y’, where both ‘x’ and ‘y’ are some sort of object and ‘f’ is an algorithm. Think of algorithms as a Coke vending machine:
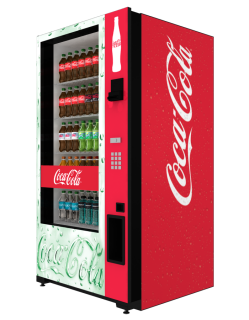
So you happen to know that when a function called ‘CokeMachine’ takes in an input of ‘1 dollar’ the output will be ‘1 Bottle of Coke’. But that is just the way this function works on a high level. If you wanted to program how this function works (i.e. work for Coca Cola), the description of the input ‘1 dollar’ wouldn’t be sufficient. 1 dollar (the assumed cost for this example) as an object could be given as 20 nickels, 4 quarters, 10 dimes, or assuming we’re not using the US dollar, many other denominations of other currencies. So before you can actually write a set of instructions on how to take money in and deliver beverages out, you must understand some assemblance of what objects with which you are dealing. This is why it is so important to first understand objects, then work on learning how to engineer algorithms.
Algorithms Reclaim Time
You can think of Algorithms as Recipes – a set of instructions that you give to the computer and it executes. This execution is what makes Computational Engineering valuable – this the computer doing work that otherwise would take up your time. What is important to remember as you go forward is that every single software you are using on a daily basis – every interaction you have with your PC, phone, or even television – breaks down to you interacting with a set of instructions that were written by a human in a language the computer could interpret. When you click “Send” on an email you had just written, it sets off a series of pre-described actions that the computer has been told, by human, to execute when this occurs. As a Computational Engineer, a large part of getting the computer to handle the mundane/repetitive tasks in your workflow will be becoming used to how to speak the computers lingo such that the computer becomes you design assistant. To be able to do this represents a quantum shift in the amount of work you can possibly do when compared to traditional, more manual methods.
Imagine you wanted to send a letter to the other side of the world without email. The algorithm for this would look like:
- Write Letter
- Put Letter in Envelope
- Put Stamp on Envelope
- Place Letter in Mailbox
But that’s just your steps, after this, the postal services algorithm kicks in, looking something like this:
- Separate letters based on country
- Send all letters to their countries’ postal service
- In the country, separate letters into their postal codes
- Send letters to each postal code
- In the postal code, separate letters into different routes
- For each route, delivery person to deliver letter
- Letter Received
Every step of this algorithm involves humans and therefore, it occurs to human timescales. You have experience that even in the modern era, the completion of this process in on the order of hours, days, and maybe even weeks. Yet, since a person has written a script that is executed behind your “Send” button, now the algorithm looks like this:
- Write Email
- Press “Send”
- Execute “Send” code
- Letter Received
All this, as you know, happens in the matter of seconds. This example is important to show the power of eliminating humans from mundane/repetitive tasks – we gain back time. Time is of course, extremely valuable! If you are an engineer of the built environment, you know that there are a lot of repetitive tasks that make up your daily work. Clearly, being able to speak to a computer and engineer algorithms is a crucial skill in the modern era.
Different Types of Algorithms
Does this mean that we are going to eliminate the need for human engineers by coding ourselves out of a job? No. As of the time of this writing, whilst machines do a great job of taking in any email you write and delivering it to another party, you would be hard stretched to have an algorithm write the email for you. The reason for this leads us to a important concept of heuristics.
Heuristic:
1) enabling a person to discover or learn something for themselves.
2) A process of trial and error to arrive at a solution.
A heuristic problem is one whose solution requires trial and error, experience, and what we would call creativity. There is no straightforward algorithm or set of rules for these types of problems. The engineering process is full of heuristic processes. One of the best examples of this is the following scene from apollo 13:
In this case, the problem was to “invent a way to fit a round peg into a square hole”. But really, this happens all the time in engineering. Next time you have and problem to solve and you can say ‘there’s no manual for this’, know that you have a heuristic problem. Also, be happy – this is a problem that for the foreseeable future, only you can solve! In fact, we should use algorithms to make sure that is where we are spending the majority of our time. For more on understanding what heuristic design is, check out www.designheuristics.com.
As you progress up the ranks of Computational Engineering, you will start to build up a knowledge of the Computer Science pyramid. Just as importantly, you will start to gain an appreciation for which tasks are heuristic and therefore, need to be done by a human and which tasks are able to be translated into an algorithm which a machine is best to carry out.
This is an extremely important skill of a Computational Engineer – the ability to dispatch tasks between humans and machines:
So the essence of being a Computational Engineer is being able to
- recognise which tasks could be carried out by the machine
- recognise which tasks are best carried out by a human
- communicate with the computer in order to have it do repetitive tasks which free humans up to do heuristic tasks
In the next post, we will learn a framework by which you can use your engineering skills to engineer useful algorithms.