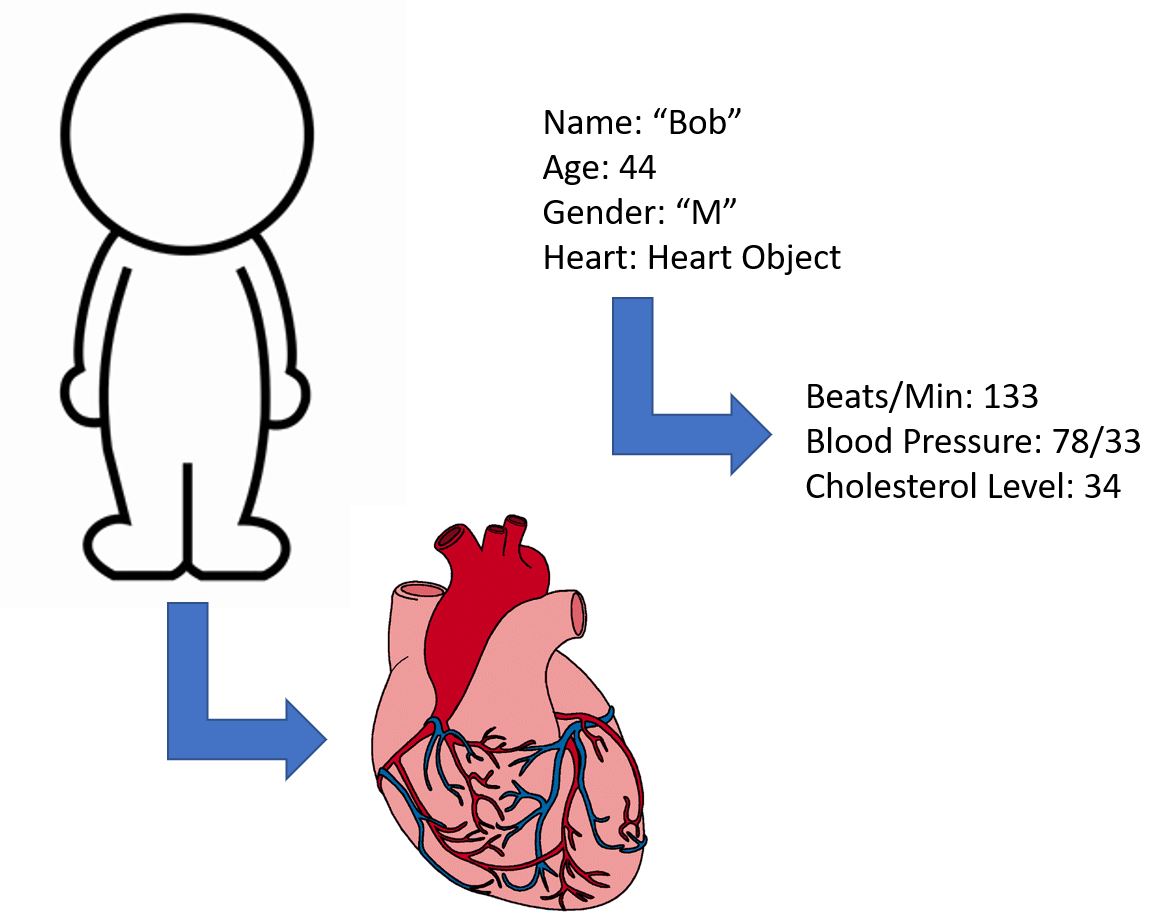
Computational Engineering 101 – Part 4: Object Oriented Programming for Computational Engineers
Why Start with Objects?
In this Part 3, we introduced the key Computational First-Principles topics with which all Computational Engineers need to be familiar – Objects and Algorithms. In this post, we will cover the very important topic of computational objects and introduce Object Oriented Programming for Computational Engineers.
In short, Objects refers to the digital definition of objects in a computer – a subject known as Objected Oriented Programming (OOP). Algorithms refer to a series of instructions which can be carried out to perform a certain task. Those of you who are mathematically inclined could also think of algorithms as functions which for a given input give you a certain output.
Most people when getting involved in Computational Engineering will start with algorithms, as they are the perceived most useful part of Computational Engineering. After all, this is where we get the computer to do work for us – isn’t that the whole point? However, as will be shown in this post, a basic knowledge of Objects is a prerequisite to learning how to develop these algorithms. Objects are the lifeblood flowing through your algorithms and ultimately to code or use these algorithms you will need to have a basic understanding of objects.
What are Objects?
As a designer of the built in environment, you are in the business of creating tangible objects. Luckily for you, there is an entire wing of computer science dedicated to representing objects using a computer – it’s called Objected Oriented Programming (OOP). We won’t get into the fine details here, but what’s important is that you understand the basics of what it means to define an object inside a computer.
A computational object object consists of properties and methods. Properties (a.k.a attributes, parameters, fields) are specific variables that are assignable to that type object. For instance, if we were defining a “Human” object, one property we may expect to would be “Height”, which could be represented as a number, such as ‘180.0’ or could be represented as text, such as “180cm”. Equally, an object’s properties could be other objects. For instance, a Human object may contain a property “Heart” which is a specific object that contains properties such as “Beats Per Minute” or “Blood Pressure”. In this situation, both of these properties of the Heart object probably are best described by numbers, although, the Heart object could just as easily have other objects as properties. As such, Objects usually are themselves hierarchical – inside of one object lies a set of properties and in each of those properties there is a set of other properties and so forth.
The Object’s methods are functions (a.k.a algorithms, routines, recipes) that are associated to the object – more on this in the next section. For instance, a Human could have a function called Blink(# of Seconds) associated to it. This function takes in a parameter called “# of seconds” as an input which makes the human object blink every “# of seconds” we input.
Let’s not get too bogged down in defining objects for now, but rather, focus in on why they are important – they are what we create! Whether its a beam, duct, pipe, window, train, sewer, line, circle, or anything else, during design, you are creating a hypothetical object that will eventually manifest itself physically in the built environment. Therefore, the closer we can model own our hypothetical objects in the computer, the close we are to using the computer as a valuable design assistant.
What do I see on the screen?
In order to do this, a critical skill you must develop is to understand there is a difference between what you see and what the computer ‘sees’. Let’s take a quick example:
What do you see in the above picture? Hopefully, you can see a Rhino window with a bunch of frowning faces. But this isn’t what the computer sees – the computer has no idea what a face is, what a frown is, what a human is, or anything of the sort. The computer’s world is processing 1’s and 0’s – that’s it. That’s a key concept to understand – anything you can describe using human languages (i.e. ‘frowning face’) is not existing to the computer as anything more than a bunch of 1’s and 0’s. The machine has zero empathy for the way you see the world. The onus is on you to have empathy with the way the machine sees the world if you want to be a Computational Engineer.
What does the computer see on the screen?
If we use the ‘list’ command in Rhino, we effectively can ask Rhino how it is interpreting what we are seeing:
Upon clicking one of the faces, we can see that first off, the outer face has nothing to do with the mouth, nor eyes. The outer part of what we would call the ‘face’ is called a ‘curve’ as seen in the first line of the ‘list’ result. In fact, there is a lot of data displayed here that is completely out of the scope of our response to the question. For instance, did you respond ‘frowning face’ or did you respond something about an alpha numeric ID like the machine did? In essence, the machine sees a bunch of objects like the one we’ve clicked and asked about. All of these objects have properties like the one shown such as ‘id’, ‘index’, ‘ON_ArcCurve’, etc. The amalgamation of these properties is meant conveniently represent the object in the machine as it is in our minds.
If you are familiar with CAD, the response Rhino (AutoCAD will do the same) is not all that surprising – you take it for granted that the computer does not speak the same language as you. From this, we can glean two important lessons.
The first lesson here is that just as equally as we must appreciate the objects we are creating from first principles, we must also respect how the computer and various engineering software represent those same objects. For the purposes of our discussion we will assume that everything, even numbers and text are nothing more than ‘objects’ to the machine.
The second important lesson here is the mechanics of the process of asking the computer what it sees. This skill will come with time and varies between softwares and user interfaces, and whilst it is important, it is more important to understand the concept of OOP, rather than getting bogged down in the mechanics of how to use software.
Objects – The Structure of Data
If you have a look at my series on the history of data in the built environment, you will see how the data has been presented differently to us humans over the years, but also, how was the data seen differently to the computer over the years. A quick synopsis of that series will be useful here:
- Turnips/physical models – Either not seen by the computer or seen as matrix pixels with associated colors (digital picture)
- “dirty smudges on paper” – Either not seen by the computer or seen as a matrix of pixels with associated colors (a scan)
- CAD – seen as a database programmatic objects – these objects are geometrical objects with associated properties such as Layer Names
- ‘Commercial’ BIM – seen as a database programmatic objects – the objects are custom objects aimed at digitally defining the object being designed and has properties that represent the properties the physical object should manifest upon its creation
As you can see, the structure of the data is becoming more and more relevant to the object which it represents as time goes forward. You can see why drawing the design used to be called ‘drafting’ whereas modern day BIM technicians would be ‘modelling’ – the data is getting more structure and rich. The key for a computational engineer is that with all this data structured in this way, it can be easily manipulated with functions to get us useful insights, create new objects, or many other useful design tasks. Computational Engineers see a design as data, and that data in large part is structured as objects. This is why Object Oriented Programming for Computational Engineers is a key computational principle to focus on first before we move into the topic of algorithms in the next post.